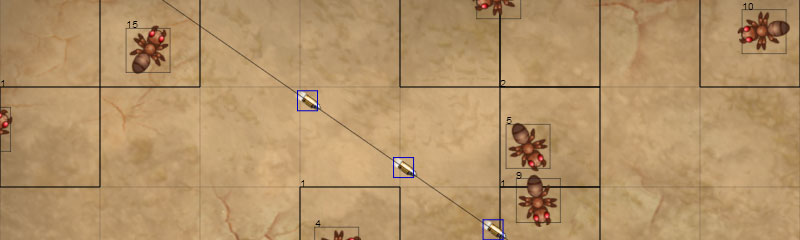
Being able to render graphics is the first step to game development. Lets start with the best source available to draw content using HTML5's Canvas element.
To commence place the html markup inside any html document.
With the markup in place we can focus on the JavaScript where all the magic happens. To initiate a 2d drawing area we will grab the canvas element and extract the 2d context.
var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d');
The context is the object that enables us to render various objects using the built in functions. We will start with how to draw a basic line.
// Draw Line context.beginPath(); context.moveTo(20, 20); context.lineTo(45, 70); context.lineWidth = 5; context.strokeStyle = "rgba(255,0,0,1)"; context.stroke();
With the beginPath(x,y) function we can start the process to render an object. The moveTo(x,y) function moves the pointer to the x and y location. To complete a line we use the lineTo() function which draws a line to the next x and y position. Finally to enable the line we use the stroke() function. We also have the capability to change the width of the line with the lineWidth value. The default color is black and can be changed with the strokeStyle value. Above this has been set to red in the RGBA value with 'A' standing for the alpha value set from zero to one where one is solid.
// Draw Circle context.beginPath(); context.arc(90, 45, 25, 0, 2*Math.PI, true); context.strokeStyle = "rgba(0, 255, 0, 1)"; context.stroke();
With the arc(x,y,radius,0,2*Math.PI) function we can also draw the classic circle. The center point is defined by the x and y. The radius is the distance from the center to the outer edge. So our circle above is 50 pixels in diameter and our circle will be green.
// Draw Rectangle context.beginPath(); context.rect(140, 20, 80, 50); context.strokeStyle = "rgba(0, 0, 255, 1)"; context.stroke(); context.fillStyle = "rgba(0, 0, 255, 0.5)"; context.fill();
Another valuable shape to draw is the rectangle. With the rect(x,y,width,height) function the x and y represents the top left point and the width and height in pixels leads to the bottom right point. With fillStyle() we can create a solid colored object and our rectangle is now filled blue with a border stroke on top of it.
// Draw Image var image = new Image(); image.onload = function() { context.drawImage(image, 160, 90); }; image.src = '/image/location/image.png';
Finally the most important form of drawing objects is the image, which is essential for creating sprites and animations. First we create an Image object and define a function which will be called when the image is completely loaded. Its important to put the onload function before you define the source of the Image object. Last and final step is to point to the file location.
Now lets take a look at all the code together. With the ability to render all these different shapes and images we can now put together a complete game.
var canvas = document.getElementById('canv'); var context = canvas.getContext('2d'); // Draw Line context.beginPath(); context.moveTo(20, 20); context.lineTo(45, 70); context.lineWidth = 5; context.strokeStyle = "rgba(255,0,0,1)"; context.stroke(); // Draw Circle context.beginPath(); context.arc(90, 45, 25, 0, 2*Math.PI, true); context.strokeStyle = "rgba(0, 255, 0, 1)"; context.stroke(); // Draw Rectangle context.beginPath(); context.rect(140, 20, 80, 50); context.strokeStyle = "rgba(0, 0, 255, 1)"; context.stroke(); context.fillStyle = "rgba(0, 0, 255, 0.5)"; context.fill(); // Draw Text context.fillStyle = "rgba(0, 0, 0, 1)"; context.fillText("Intro to HTML5 Canvas", 100, 250); // Draw Image var image = new Image(); image.onload = function() { context.drawImage(image, 160, 90); }; image.src = '/image/location/image.png';